Random & Perlin Noise
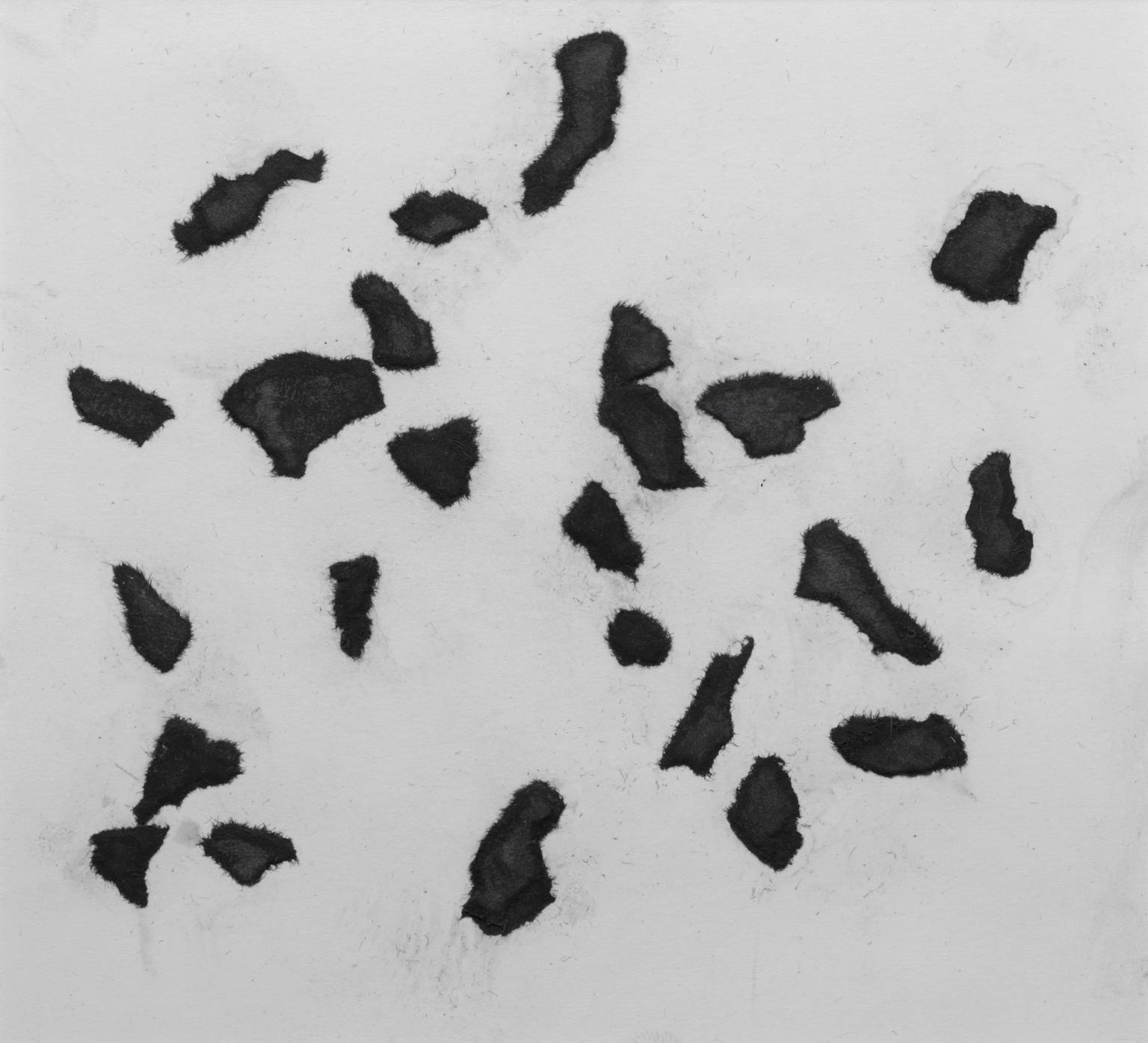
“A straight horizontal thread one meter long falls from a height of one meter onto a horizontal plane twisting as it pleases and creates a new image of the unit of length.” Marcel Duchamp, 3 Standard Stoppages, Paris 1913-14.
Duchamp later said that 3 Standard Stoppages opened the way “to escape from those traditional methods of expression long associated with art,” such as what Duchamp called “retinal painting,” art designed for the luxuriance of the eye. This required formal intelligence and a skillful hand on the part of the artist. The Stoppages, on the other hand, depended on chance—which, paradoxically, they at the same time fixed and “standardized.” (Duchamp used the phrase “canned chance.”)Publication excerpt from The Museum of Modern Art, MoMA Highlights, New York: The Museum of Modern Art. MoMa
Chance (random) is not a new concept. Many artists sought ways to introduce it into their creative process. From Jean Arp to Marcel Duchamp, from Jackson Pollock to John Cage, it is present.
Computers (in theory) are excellent at executing commands in such precision that we can predict its results if we ignore for a moment glitches, bugs, human errors, and unexpected interruptions.
There are many methods developed over the years for introducing randomness into the software. Some are predictably random, others are truly random. Below, I'll explain a few random generators that exist in p5.js.
Random
random()
generates a random float that is between 0 and 1. You can pass a single argument or multiply it to have a random number that is between 0 and x.
// A random float between 0 and 1
let r1 = random();
// A random float between 0 and 100
let r2 = random() * 100;
// A random float between 0 and 100
let r3 = random(100);
It is also possible to select a random item from an array.
let fruits = ["orange", "mango", "banana", "kiwi"]
// A random element
let r1 = random(fruits);
RandomSeed
If you want to get the same random numbers each time you run your sketch, you can use randomSeed(seed)
. Seed parameter you provide initializes a pseudo-random number generator. However, seed should not be random itself, to be able to reproduce pseudo-randoms.
Perlin Noise
Perlin noise is developed by Ken Perlin in 1983 to generate a smooth, non "machine-like" sequence of random numbers to produce textures. Number(s) given as parameter determines the location in noise space. The increment amount changes the smoothness (or zoom level) of the output.
for (let i=0; i<200; i++) {
// Increments of 1
line(i, 0, i, noise(1 * i) * -50);
// Increments of 0.1
line(i, 0, i, 100 + noise(0.1 * i) * -50);
// Increments of 0.01
line(i, 0, i, 200 + noise(0.01 * i) * -50);
// Increments of 0.001
line(i, 0, i, 300 + noise(0.001 * i) * -50);
}
noise()
accepts 1, 2, or 3 parameters, which corresponds to output in 1D, 2D, or 3D.
There is a good article about using Perlin noise to create flow fields by Manohar Vanga (Sighack):
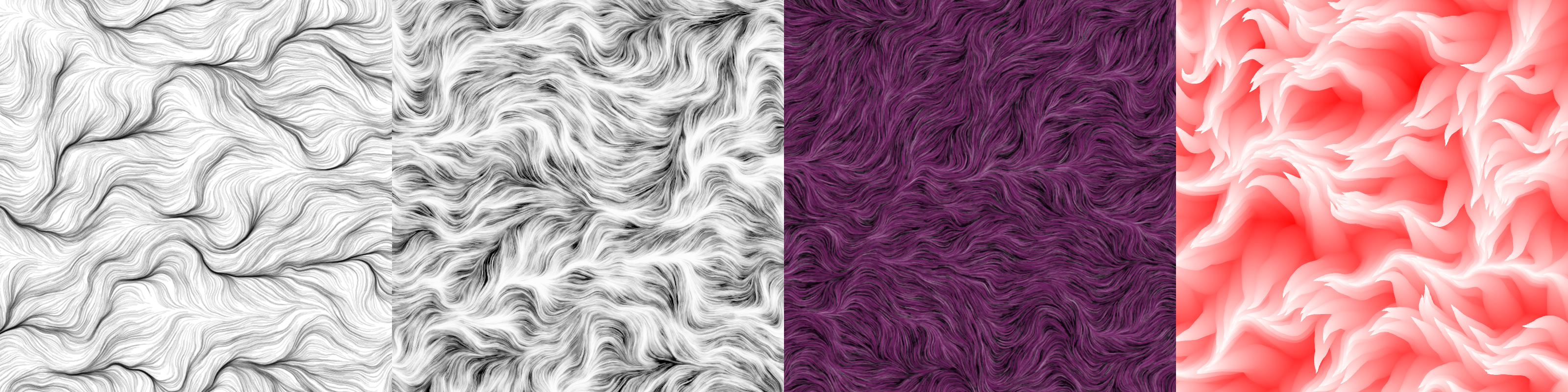
Noise can be used in many creative ways: